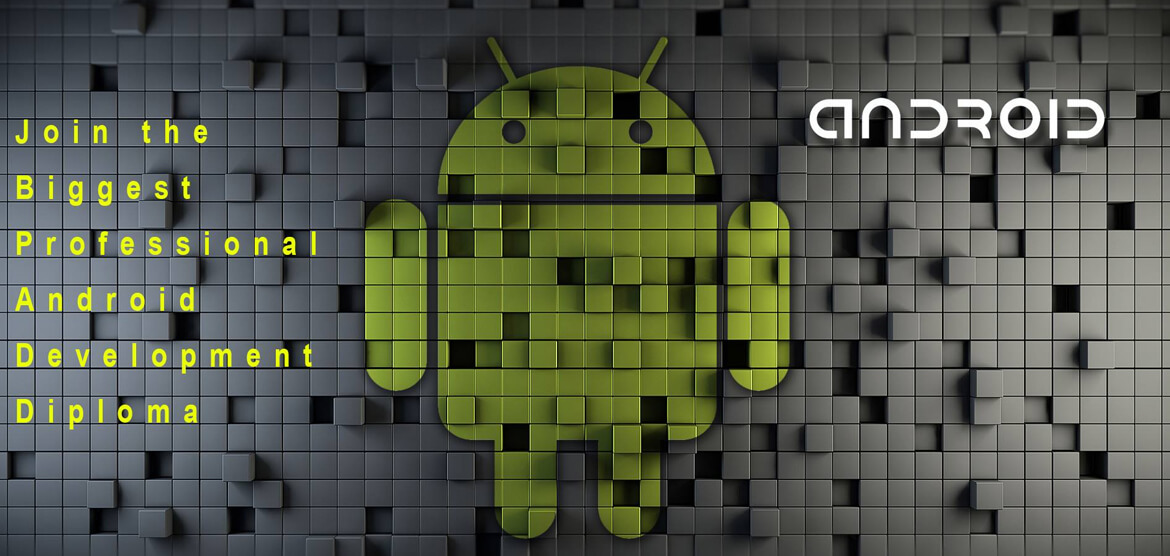
Android Course
Part 1: Concepts of JAVA
Principles of java
- What's Java ?
- Java history.
- Java Bytecode.
- Java virtual machine.
- JRE(Java runtime environment).
- JDK (Java Development kit).
- SDK(Software Development Kit).
- API(Application Programming Interfaces).
- Variables.
- Data Types.
- Strings.
- Input-output.
- Comments.
- Math Operations.
- Conditional statement (if- switch).
- Looping.
- Arrays.
- ArrayList.
- Exceptions.
- Streams (input stream- output stream).
- Casting.
OOP
- Class.
- Object.
- Member Variable.
- Member method.
- Constructor.
- Access Control Modifiers.
- Inheritance.
- Polymorphism.
- Interfaces.
- Abstraction.
Workshop #1
- Implementing OOP scenario using Java.
Part 2: Android
Introduction to Android
- What’s Android?
- Why Android.
- Android Market.
- Android Versions.
- what’s new Android.
Android Architecture
- Android Features.
- Android Stack.
- Android Architecture layer.
Tools
- SDK (software development kit).
- JDK (java development kit).
- IDE (integrated development environment.
Android Studio
- Setup Android Studio.
- Android Manifest.
- Important Folders.
- Important Buttons.
- logcat
- Emulator and genymotion.
Android Components
- activities and services
- Broadcast Receivers.
- Content Providers.
Building Application UI
- Material design concept.
- Layouts
- Layouts type
- attributes
- View class.
Activities
- Life Cycle.
- Call Back.
- Methods Interacting with UI.
- Resources.
Intents
- Explicit Intents.
- Implicit Intent.
- Intent Filter.
listView
- adapter.
- listviews
- custom views.
- RecyclerView.
Networking
- threads
- Async tasks
- Volley library.
- JSON Parsing.
Data Storing
- Shared preferences.
- Internal storage.
- External storage.
- sqlite database.
Services
- Overview about services in Android and its life cycle.
- Implementing a Service.
- Implementing Intent service.
Fragments
- static and dynamic
- Android Fragments Tabs.
- Android Navigation Drawer.
Camera
- Working with camera.
Multimedia in Android
- Simple Media Player APP.
- Simple video playback.
Menus
- Context Menu.
- Popup Menu.
- Option Menus.
Notifications
- Notification properties.
- Attach Actions to notification.
- Pending Intent.
Maps and Locations
- Working with Google Maps.
- Finding current location and listening for changes in location.
- Working with GPS.
Advanced Topics
Threads
- UI(Main) thread versus worker thread.
- Loopers, handlers, and event flow from system.
- Writing multi threading programs.
Async tasks
- Writing threading programs using Async tasks..
- Updating UI from worker threads..
Data parsing
- XML and JSON..
Reading and writing phone storage
Workshop #2
- Groups of students.
- 2 days of work.
- Implementing full app.
- Uploading app to playstore.