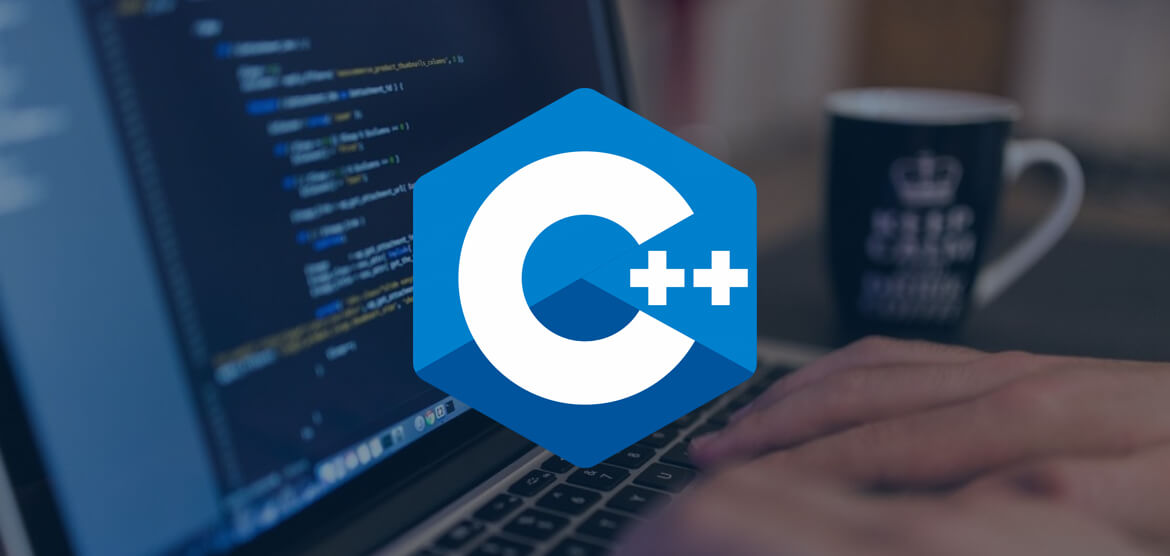
C++/OOP
Module one:-
- Introduction and Principles
- What is programming language?
- How the programs run?
- Output functions.
- Variables and Data types.
- Read value from user and process it.
- Mathematical basics.
- Conditional statements
- If-else.
- Dangling-else Problem.
- switch.
- Repetition statements
- For, while, do-while.
- Counter-Controlled Repetition.
- Sentinel-Controlled Repetition.
- Break and continue Statements.
- Nested Control Statements.
- Functions
- Function Prototypes, built in functions and user defined functions.
- References and Reference Parameters, Scope Rules.
- Function overloading, and Function Templates.
- Inline Functions and recursive functions.
- Math Library Functions.
- Arrays
- Introduction to arrays.
- Passing arrays to functions.
- Searching Arrays with Linear Search.
- 2-D arrays.
- Multidimensional arrays.
Module Two:-
- Pointers
- Call by reference, Call by value, and const member functions.
- Pointer Variable Declarations and Initialization.
- Pointer Operators.
- Passing Arguments to Functions by Reference with Pointers.
- Relationship between Pointers and Arrays.
- Pointer-Based String Processing.
- Structures, Union and Enumeration
- Define struct.
- Use structs with functions.
- Union.
- Enumeration.
- Files Streaming
- Files and Streams.
- Creating a Sequential File, Create, read and update file.
- Random-Access Files.
- Writing Data Randomly to a Random-Access File.
- Memory Allocation and Bitwise Operators
- Dynamic Memory allocation.
- Bitwise Operators.
- Function with dynamic numbers of arguments.
Module Three:-
- OOP Concepts
- Classes & Objects in detail.
- Access Modifiers.
- Constructor & Destructor.
- Inheritance.
- Polymorphism.
- Overloading.
Module Four:-
- Problem solving practice on ( Codeforces , hackerRank , codility ).
- Big O notation.
- Introduction About Algorithms && Data Structure.
Module Five:-
- Project
- Group of 2-3 persons select an idea.
- The lecturer discusses the code with you.